Testing Laravel apps with multiple domains
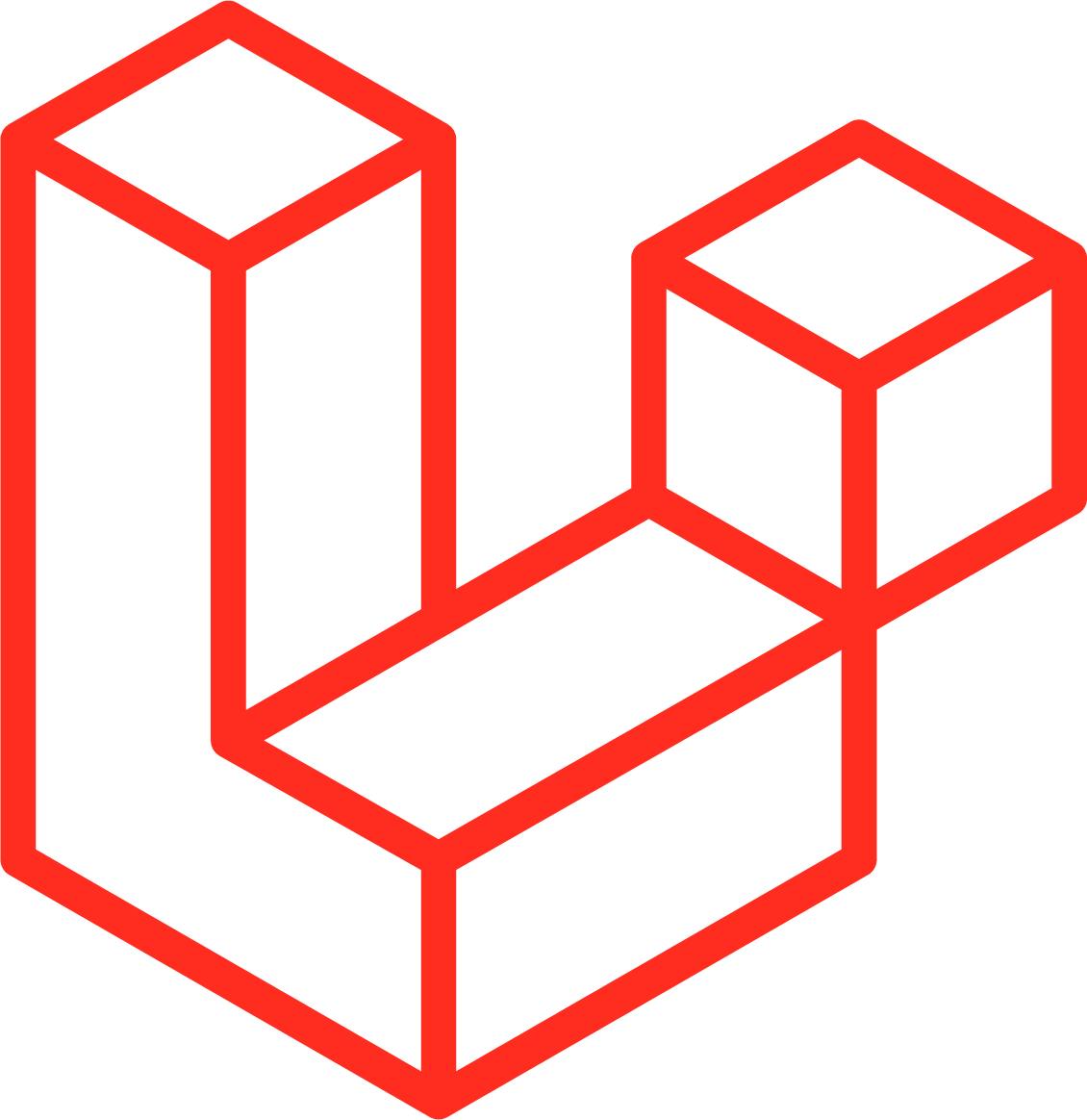
When testing Laravel applications that use multiple domains tests get tricky. Imagine an application with the following structure:
- API: api.domain.com
- Backend: manage.domain.com
- Client domains: client1domain.com,client2domain.com, etc..
Route::domain(config('app.domains.api'))->group(function() {
Route::get('/', 'Api\ApiIndexController@index')->as('api.index');
});
Route::domain(config('app.domains.backend'))->group(function() {
Route::get('/', 'Backend\BackendIndexController@index')->as('backend.index');
});
// fallback to client domains
Route::group(function() {
Route::get('/', 'Sites\SitesIndexController@index')->as('site.index');
});
Now let’s see how we would go about testing the routes within each domain.
namespace Tests\Feature\Api;
use Tests\TestCase;
class ApiDomainTest extends TestCase
{
function setUp(): void
{
parent::setUp();
config(['app.url' => "http://${config('app.domains.api')}"]);
}
public function testApiIndex()
{
$response = $this->json('GET', route('api.index'));
$response->assertStatus(200)->assertSeeText('API index.');
}
}
namespace Tests\Feature\Backend;
use Tests\TestCase;
class BackendDomainTest extends TestCase
{
function setUp(): void
{
parent::setUp();
config(['app.url' => "https://${config('app.domains.backend')}"]);
}
public function testBackendApiIndex()
{
$response = $this->json('GET', route('backend.index'));
$response->assertStatus(200)->assertSeeText('Backend index.');
}
}
namespace Tests\Feature\Site;
use Tests\TestCase;
class SiteDomainsTest extends TestCase
{
public function testClient1SiteIndex()
{
config(['app.url' => "http://clientdomain1.com"]);
$response = $this->json('GET', route('site.index'));
$response->assertStatus(200)->assertSeeText('This is client site 1 index.');
}
public function testClient1SiteIndex()
{
config(['app.url' => "http://clientdomain2.com"]);
$response = $this->json('GET', route('site.index'));
$response->assertStatus(200)->assertSeeText('This is client site 2 index.');
}
}